编程免不了要写配置文件,怎么写配置也是一门学问。YAML是专门用来写配置文件的语言,非常简洁和强大,远比 JSON 格式方便。
一、基础
标准输入输出
- input函数,从默认标准输入键盘读取一行文本
- print函数,将内容输出到默认标准输出设备屏幕
文件I/O
- open函数,Python内置方法,打开一个文件(根据情况指定打开模式),创建并返回一个file对象,常用模式:
- t:文本模式(默认)。
+
:打开一个文件进行更新(可读可写)。- r:以只读方式打开文件。文件的指针将会放在文件的开头。这是默认模式。
- r+:打开一个文件用于读写。文件指针将会放在文件的开头。
- w:打开一个文件只用于写入。
- 如果该文件已存在则打开文件,并从开头开始编辑,即原有内容会被删除。
- 如果该文件不存在,创建新文件。
- w+:打开一个文件用于读写。
- 如果该文件已存在则打开文件,并从开头开始编辑,即原有内容会被删除。
- 如果该文件不存在,创建新文件。
- a:打开一个文件用于追加。
- 如果该文件已存在,文件指针将会放在文件的结尾,也就是说新的内容将会被写入到已有内容之后。
- 如果该文件不存在,创建新文件进行写入。
- a+:打开一个文件用于读写。
- 如果该文件已存在,文件指针将会放在文件的结尾,也就是说新的内容将会被写入到已有内容之后。
- 如果该文件不存在,创建新文件用于读写。
- read函数,file对象方法,从一个打开的文件中读取一个字符串。
- write函数,file对象方法,将任何字符串写入一个打开的文件。
- close函数,file对象方法,刷新缓冲区里任何还没写入的信息,并关闭该文件
- open函数,Python内置方法,打开一个文件(根据情况指定打开模式),创建并返回一个file对象,常用模式:
file对象
- file属性
- file.closed:返回true如果文件已被关闭,否则返回false。
- file.mode:返回被打开文件的访问模式。
- file.name:返回文件的名称。
- file.softspace:如果用print输出后必须跟一个空格符,则返回false。
- file方法
- file.close():关闭文件,关闭后文件不能再进行读写操作。
- file.flush():刷新文件内部缓冲,直接把内部缓冲区的数据立刻写入文件,而不是被动的等待输出缓冲区写入。
- file.fileno():返回一个整型的文件描述符(
file descriptor FD
整型),可以用在如os模块的read方法等一些底层操作上。 - file.isatty():如果文件连接到一个终端设备返回True,否则返回False。
- file.next():返回文件下一行。
- file.read([size]):从文件读取指定的字节数,如果未给定或为负则读取所有。
- file.readline([size]):读取整行,包括
\n
字符。 - file.readlines([sizeint]):读取所有行并返回列表,若给定sizeint>0,则是设置一次读多少字节,这是为了减轻读取压力。
- file.seek(offset[, whence]):设置文件当前位置
- file.tell():返回文件当前位置。
- file.truncate([size]):截取文件,截取的字节通过size指定,默认为当前文件位置。
- file.write(str):将字符串写入文件,返回的是写入的字符长度。
- file.writelines(sequence):向文件写入一个序列字符串列表,如果需要换行则要自己加入每行的换行符。
- file属性
二、使用
- 标准输入输出,demo1.py
- 代码
1 | # coding=utf-8 |
- 运行
python3.8 demo1.py
- 文件I/O,demo2.py
- 代码
1 | # -*- coding: UTF-8 -*- |
- 运行
python3.8 demo2.py
三、读写csv-xls-xlsx文件
读写csv
- reader/writer方式
1
2
3
4
5
6
7
8
9
10
11
12
13
14import csv
rFile = open("test.csv", "r")
wFile = open("new.csv", "w")
reader = csv.reader(rFile)
writer = csv.writer(wFile)
for item in reader:
header = []
if reader.line_num == 1:
header = item
writer.writerow(header)
else:
writer.writerow(item)
wFile.close()
rFile.close()- DictReader/DictWriter方式
1
2
3
4
5
6
7
8
9
10
11
12
13import csv
rows = [
{'name': 'tom', 'age': 10, },
{'name': 'tim', 'age': 11, }
]
fieldnames = ['name','age'] #定义表头字段
cr = csv.DictWriter(open('haha.csv','w'),fieldnames = fieldnames)
cr.writeheader()
cr.writerows(rows)
reader = csv.DictReader(open('haha.csv','r'))
for line in reader:
print(line)读写xls
pandas方式
pip install pandas
- demo
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20import csv
import os
import pandas as pd
import xlrd
import xlwt
import openpyxl
from xlutils.copy import copy
#pandas依赖xlrd库
#方式一
read = pd.ExcelFile('test.xlsx')
data1 = read.parse('one')
#方式二
data2 = pd.read_excel('test.xlsx',sheet_name='one')
#无则新建
writer = pd.ExcelWriter('test_new.xlsx')
data1.to_excel(writer, sheet_name='mysheet', index=False)
data2.to_excel(writer, sheet_name='another', index=False)
writer.save()
os._exit(0)xlrd/xlwt方式
- pip install xlrd xlrw
- demo
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58import csv
import os
import pandas as pd
import xlrd
import xlwt
import openpyxl
from xlutils.copy import copy
#xlwt/xlrd方式,支持xls和xlsx
def write_xls(path, sheet_name, value):
index = len(value)
workbook = xlwt.Workbook()
sheet = workbook.add_sheet(sheet_name)
for i in range(0, index):
for j in range(0, len(value[i])):
sheet.write(i, j, value[i][j])
workbook.save(path)
print('xls写入成功')
def write_xls_append(path, value):
index = len(value)
workbook = xlrd.open_workbook(path)
sheets = workbook.sheet_names()
worksheet = workbook.sheet_by_name(sheets[0])
rows_old = worksheet.nrows
new_workbook = copy(workbook)
new_worksheet = new_workbook.get_sheet(0)
for i in range(0, index):
for j in range(0, len(value[i])):
new_worksheet.write(i+rows_old, j, value[i][j])
new_workbook.save(path)
print("xls追加成功")
def read_xls(path):
workbook = xlrd.open_workbook(path)
sheets = workbook.sheet_names()
worksheet = workbook.sheet_by_name(sheets[0])
for i in range(0, worksheet.nrows):
for j in range(0, worksheet.ncols):
print(worksheet.cell_value(i, j), "\t", end="")
book_name_xls = 'xlwt.xlsx'
#book_name_xls = 'xlwt.xls'
sheet_name_xls = 'sheet_one'
value_title = [["姓名", "性别"]]
value1 = [["张三", "男"]]
value2 = [["李四", "男"]]
write_xls(book_name_xls, sheet_name_xls, value_title)
write_xls_append(book_name_xls, value1)
write_xls_append(book_name_xls, value2)
read_xls(book_name_xls)
* openpyxl方式
* pip install openpyxl
* demo
def write_xlsx(path, sheet_name, value):
index = len(value)
workbook = openpyxl.Workbook()
sheet = workbook.active
sheet.title = sheet_name
for i in range(0, index):for j in range(0, len(value[i])): sheet.cell(row=i+1, column=j+1, value=str(value[i][j]))
workbook.save(path)
print(“xlsx格式表格写入数据成功!”)
def read_xlsx(path, sheet_name):
workbook = openpyxl.load_workbook(path)
sheet = workbook[sheet_name]
for row in sheet.rows:
for cell in row:
print(cell.value, "\t", end="")
name = 'openpyxl.xlsx'
sheet = 'sheet1'
value = [["姓名", "性别"],
["张胜男", "女"],
["李思思", "男"],
["李宇春", "女"]
]
write_xlsx(name, sheet, value)
read_xlsx(name, sheet)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
3. 参考
* [CSV File Reading and Writing](https://docs.python.org/3/library/csv.html)
* [Python标准库](https://docs.python.org/zh-cn/3/library/index.html)
* [Pandas文档](https://pandas.pydata.org/pandas-docs/stable/reference/api/pandas.ExcelWriter.html)
## 四、多文件夹批量文件操作
>有了宝宝以后,每个宝爸宝妈手机里基本都是自家宝宝的各种靓照,时间久了手机内存自然是不够用的。此时我们可以选择将照片备份在百度云盘等应用中,不过为了以防万一,可以在本地电脑做一下备份。第一次备份时没有做过多设置(可以设置存储格式),最终导入电脑的时候是以拍摄日期为文件夹分开存储的,导致后期查看不方便,所以准备将多个文件夹的内容分别取出来放到一个文件夹下。
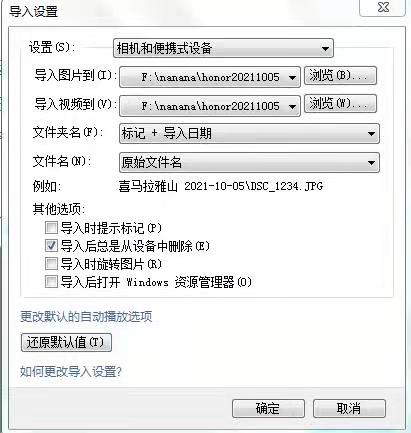
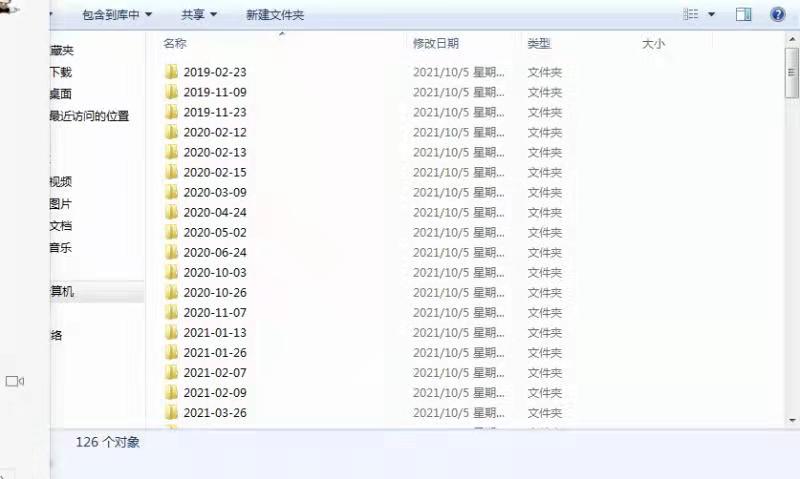
1. 环境
* Win7
* Python2.6.2
2. 脚本`multiMove.py`
#!/usr/bin/python
-- coding: UTF-8 --
import os, sys, shutil
#path = “F:/nanana/huawei20210920”
path = “F:/nanana/oppo20210920”
#path = “D:\downloads”
dirs = os.listdir(path)
‘’’
shutil.copy(“F:/nanana/a.txt”,”F:/nanana/huawei”)
sys.exit()
‘’’
for dir in dirs:
#print dir
tmpPath = path + ‘/‘ + dir
print tmpPath
tmpDir = os.listdir(tmpPath)
for file in tmpDir:
try:
tmp = path + ‘/‘ + dir + ‘/‘ + file
print tmp
if os.path.isfile(tmp):
#shutil.copy(tmp, “F:/nanana/huawei”)
shutil.copy(tmp, “F:/nanana/oppo”)
except Exception as ex:
print(“error info:” + str(ex))
3. 运行`python multiMove.py`
4. TODO
* 路径可配置可输入
* 支持自定义参数
* 打包为可执行文件
5. [参考](https://docs.python.org/zh-cn/2.7/library/index.html)